Removing a recursion in Python, part 2
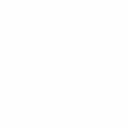
Good day all, before we get into the continuation of the previous episode, a few bookkeeping notes.
First, congratulations to the Python core developers on successfully choosing a new governance model. People who follow Python know that the Benevolent Dictator For Life has stepped down after 30 years of service, which led to a great many questions about how to best run an open-source, community-driven programming language. I'm really looking forward to seeing how the new governance model works and evolves.
Second, there were a number of good comments on my last episode. Several people noted that the given problem could be turned into a much simpler iterative form than the one I gave. That's certainly true! Remember, the problem that inspired this series was not a particularly interesting or important problem, it was just a problem where I realized that I could demonstrate the general method of removing a recursion. The general method is not the best method for all possible specific cases. Also, some commenters noted that there are other general ways to remove a recursion; indeed there are, and some of them do make for more readable code.
Third, have a festive holiday season all, and a happy New Year; we'll pick up with more fabulous adventures in 2019!
Today I want to look at a rather different technique for removing a recursion, called continuation passing style. Long-time readers will recall that I discussed this technique in Javascript back in the early days of this blog, and that a variation on CPS is the mechanism that underlies async/await in C#.
In our example of last time we made a stack of "afters" - each "after" is a method that takes the return value of the "previous" recursive call, and computes the result of the "current" recursive call, which then becomes the argument to the "next" after, and so on. The "continuation" in "continuation passing style" just means "what happens after"; the code that happens after a bit of code is the continuation of that code in your program.
Put another way: in our original recursive program, we return and that returned value is then passed directly to the continuation of the recursive call. A return is logically the same as a method call to the continuation.
The idea of continuation passing style is, you guessed it, we're going to pass in the continuation as an argument, and then instead of returning, we're going to call the continuation.
Let's suppose we again have this general form of a one-recursion recursive function:
def f(n): if is_base_case(n): return base_case_value argument = get_argument(n) after = get_after(n) return after(f(argument))
How might we turn this into the equivalent program in CPS? We have two returns; both of them must turn into calls to the continuation. The first one is straightforward:
#we're now passing in continuation c, a function of one argumentdef f(n, c): if is_base_case(n): c(base_case_value) return
Easy peasy. What about the non-base case? Well, what were we going to do in the original program? We were going to (1) get the arguments, (2) get the "after" - the continuation of the recursive call, (3) do the recursive call, (4) call "after", its continuation, and (5) return the computed value to whatever the continuation of f is.
We're going to do all the same things, except that when we do step (3) now we need to pass in a continuation that does steps 4 and 5:
argument = get_argument(n) after = get_after(n) f(argument, lambda x: c(after(x)))
Hey, that is so easy! What do we do after the recursive call? Well, we call after with the value returned by the recursive call. But now that value is going to be passed to the recursive call's continuation function, so it just goes into x. What happens after that? Well, whatever was going to happen next, and that's in c, so it needs to be called, and we're done.
Let's try it out. Previously we would have said
print(f(100))
but now we have to pass in what happens after f(100). Well, what happens is, the value gets printed!
f(100, print)
and we're done.
So" big deal. The function is still recursive. Why is this interesting? Because the function is now tail recursive! A tail-recursive method is a recursive method where the last thing that happens is the recursive call, and those methods are very easy to remove the recursion, because they can easily be turned into a loop:
# This is wrong!def f(n, c): while True: if is_base_case(n): c(base_case_value) return argument = get_argument(n) after = get_after(n) n = argument c = lambda x: c(after(x))
The naive transformation of the code above into a loop is wrong; do you see why? Give it some thought and then read on.
.
.
.
.
.
.
.
Hopefully you figured it out; the lambda is closed over variables c and after, which means that when they change, they change in every implementation of the lambda. In Python, as in C#, VB, JavaScript and many other languages, lambdas close over variables, not the values the variables had when the lambda was created.
We can work around this though by making a factory that closes over the values:
def continuation_factory(c, after) return lambda x: c(after(x))# This is wrong too!def f(n, c): while True: if is_base_case(n): c(base_case_value) return argument = get_argument(n) after = get_after(n) n = argument c = continuation_factory(c, after)
It sure looks like we've correctly removed the recursion here, by turning it into a loop. But this is not right either! Again, give it some thought; this one is more subtle.
.
.
.
.
.
.
The problem we started with was that a recursive algorithm is blowing the stack. We've turned this into an iterative algorithm - there's no recursive call at all here! We just sit in a loop updating local variables.
The question though is - what happens when the final continuation is called, in the base case? What does that continuation do? Well, it calls its after, and then it calls its continuation. What does that continuation do? Same thing.
All we've done here is moved the recursive control flow into a collection of function objects that we've built up iteratively, and calling that thing is still going to blow the stack. So we haven't actually solved the problem.
Or" have we?
What we can do here is add one more level of indirection, and that will solve the problem. (This solves every problem in computer programming except one problem; do you know what that problem is?)
What we'll do is we'll change the contract of f so that it is no longer "I am void-returning and will call my continuation when I'm done". We will change it to "I will return a function that, when it is called, calls my continuation. And furthermore, my continuation will do the same."
That sounds a little tricky but really its not. Again, let's reason it through. What does the base case have to do? It has to return a function which, when called, calls my continuation. But my continuation already meets that requirement by assumption:
def f(n, c): if is_base_case(n): return c(base_case_value)
What about the recursive case? We need to return a function, which when called, executes the recursion. The continuation of that call needs to be a function that takes a value and returns a function that when called executes the continuation on that value. We know how to do that:
argument = get_argument(n) after = get_after(n) return lambda : f(argument, lambda x: lambda: c(after(x)))
OK, so how does this help? We can now move the loop into a helper function:
def trampoline(f, n, c): t = f(n, c) while t != None: t = t()
And call it:
trampoline(f, 3, print)
And holy goodness it works.
Follow along what happens here. Here's the call sequence with indentation showing stack depth:
trampoline(f, 3, print) f(3, print)
What does this call return? It effectively returns
lambda:
f(2, lambda x:
lambda:
print(min_distance(x))
so that's the new value of t.
That's not None, so we call t(), which calls:
f(2, lambda x: lambda: print(min_distance(x))
What does that thing do? It immediately returns this mess: (I'll indent it to make it more clear, but this is not necessarily legal Python.)
lambda: f(1, lambda x: lambda: (lambda x: lambda: print(min_distance(x)))(add_one(x))
So that's the new value of t. It's not None, so we invoke it. That calls:
f(1, lambda x: lambda: (lambda x: lambda: print(min_distance(x)))(add_one(x))
Now we're in the base case, so we call the continuation, substituting 0 for x. It returns:
lambda: (lambda x: lambda: print(min_distance(x)))(add_one(0))
So that's the new value of t. It's not None, so we invoke it.
That calls add_one(0) and gets 1. It then passes 1 for x in the middle lambda. That thing returns:
lambda: print(min_distance(1))
So that's the new value of t. It's not None, so we invoke it. And that calls
print(min_distance(1))
Which prints out the correct answer, print returns None, and the loop stops.
Notice what happened there. The stack never got more than two deep because every call returned a function that said what to do next to the loop, rather than calling the function.
If this sounds familiar, it should. Basically what we're doing here is making a very simple work queue. Every time we "enqueue" a job, it is immediately dequeued, and the only thing the job does is enqueues the next job by returning a lambda to the trampoline, which sticks it in its "queue", the variable t.
We break the problem up into little pieces, and make each piece responsible for saying what the next piece is.
Now, you'll notice that we end up with arbitrarily deep nested lambdas, just as we ended up in the previous technique with an arbitrarily deep queue. Essentially what we've done here is moved the workflow description from an explicit list into a network of nested lambdas, but unlike before, this time we've done a little trick to avoid those lambdas ever calling each other in a manner that increases the stack depth.
Once you see this pattern of "break it up into pieces and describe a workflow that coordinates execution of the pieces", you start to see it everywhere. This is how Windows works; each window has a queue of messages, and messages can represent portions of a workflow. When a portion of a workflow wishes to say what the next portion is, it posts a message to the queue, and it runs later. This is how async await works - again, we break up the workflow into pieces, and each await is the boundary of a piece. It's how generators work, where each yield is the boundary, and so on. Of course they don't actually use trampolines like this, but they could.
The key thing to understand here is the notion of continuation. Once you realize that you can treat continuations as objects that can be manipulated by the program, any control flow becomes possible. Want to implement your own try-catch? try-catch is just a workflow where every step has two continuations: the normal continuation and the exceptional continuation. When there's an exception, you branch to the exceptional continuation instead of the regular continuation. And so on.
The question here was again, how do we eliminate an out-of-stack caused by a deep recursion in general. I've shown that any recursive method of the form
def f(n): if is_base_case(n): return base_case_value argument = get_argument(n) after = get_after(n) return after(f(argument))...print(f(10))
can be rewritten as:
def f(n, c): if is_base_case(n): return c(base_case_value) argument = get_argument(n) after = get_after(n) return lambda : f(argument, lambda x: lambda: c(after(x)))...trampoline(f, 10, print)
and that the "recursive" method will now use only a very small, fixed amount of stack.
Of course you probably would not actually make this transformation yourself; there are libraries in Python that can do it for you. But it is certainly character-building to understand how these mechanisms work.