What’s up with TimeZoneInfo on .NET 6? (Part 2)
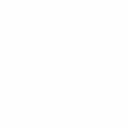
In part 1, we ended up with a lot of test data specified in a text file, but without working tests - and with a conundrum as to how we'd test the .NET Core 3.1 data which requires additional information about the hidden" AdjustmentRule.BaseUtcOffsetDelta property.
As with the previous blog post, this one is fairly stream-of-consciousness - you'll see me changing my mind and spotting earlier mistakes as I go along. It's not about trying to give advice - it's intended for anyone who is interested in my thought process. If that's not the kind of material you enjoy, I suggest you skip this post.
Abstracting platform differencesWell, time does wonders, and an answer to making most of the code agnostic to whether it's running under .NET 6 or not now seems obvious: I can introduce my own class which is closer to the .NET 6 AdjustmentRule class, in terms of accessible properties. As it happens, a property of StandardOffset for the rule makes my code simpler than adding TimeZoneInfo.BaseUtcOffset and AdjustmentRule.BaseUtcOffsetDelta together every time I need it. But fundamentally I can put all the information I need into one class, and populate that class appropriately (without even needing a TimeZoneInfo) for tests, and use the TimeZoneInfo where necessary in the production code. (We have plenty of tests that use the actual TimeZoneInfo - but using just data from adjustment rules makes it easy to experiment with the Unix representation while on Windows.)
That means adding some derived data to our text file for .NET Core 3.1 - basically working out what the AdjustmentRule.BaseUtcOffsetDelta would be for that rule. We can do that by finding one instant in time within the rule, asking the TimeZoneInfo whether that instant is in daylight savings, and then comparing the prediction of zone base UTC offset and maybe rule daylight savings" with the actual UTC offset.
With that in place, we get most of the way to passing tests - at least for the fixed data we're loading from the text file.
Hidden dataThere's one problem though: Europe/Dublin in 1960. We have this adjustment rule in both .NET Core 3.1 and .NET 6:
1960-04-10 - 1960-10-02: Daylight delta: +00; DST starts April 10 at 03:00:00 and ends October 02 at 02:59:59.999
Now I know that should actually be treated as daylight savings of 1 hour, standard offset of UTC+0". The TimeZoneInfo knows that as well - if you ask for the UTC offset at (say) June 1st 1960, you the correct answer of UTC+1, and if you ask the TimeZoneInfo whether it's in daylight savings or not, it returns true... but in most rules, a daylight delta" of 0 means this isn't in daylight time".
I believe this is basically a problem with the conversion from the internal rules to the publicly-available rules which loses some hidden" bits of information. But basically it means that when I create my standardized adjustment rule, I need to provide some extra information. That's annoying in terms of specifying yet more data in the text file, but it's doable.
Given that the Unix rules and the Windows rules are really quite different, and I've already got separate paths for them (and everything still seems to be working on Windows), at this point I think it's worth only using the enhanced" adjustment rule code for Unix. That has the helpful property that we don't need different ways of identifying the first instant in an adjustment rule: for Unix, you always use the daylight saving transition time of day; for Windows you never do.
At this point, I'm actually rethinking my strategy of introduce a new type". It's got so much more than I really wanted it to have, I think I'm just going to split what was originally one method into two:
// Hard to test: needs a time zoneinternal static BclAdjustmentRule FromUnixAdjustmentRule(TimeZoneInfo zone, TimeZoneInfo.AdjustmentRule rule)... becomes ...// Same signature as before, but now it just extracts appropriate information and calls the one below.internal static BclAdjustmentRule FromUnixAdjustmentRule(TimeZoneInfo zone, TimeZoneInfo.AdjustmentRule rule)// Easier to test with data from a text fileinternal static BclAdjustmentRule FromUnixAdjustmentRule(TimeZoneInfo.AdjustmentRule rule, string standardName, string daylightName, TimeSpan zoneStandardOffset, TimeSpan ruleStandardOffset, bool forceDaylightSavings)
The unit tests that I'm trying to get to pass with just rule data just need to call into the second method. The production code (tested in other unit tests, but only on the right" system) will call the first method.
(In the end, it turns out it's easier to make the second method return a ZoneInterval rather than a BclAdjustmentRule, but the idea is the same.)
Are we nearly there yet?At this point, I wish I'd paid slightly more attention while changing the code... because the code that did work for America/Sao_Paulo in 2018 is now failing for .NET 6. I can see why it's failing now - I'm not quite so sure why it worked a few hours before.
The problem is in this pair of adjustment rules:
2017-10-15 - 2017-12-31: Daylight delta: +01; DST starts October 15 at 00:00:00 and ends December 31 at 23:59:59.9992018-01-01 - 2018-02-17: Daylight delta: +01; DST starts January 01 at 00:00:00 and ends February 17 at 23:59:59.999
These should effectively be merged: the end of the first rule should be the start of the second rule. In most rules, we can treat the rule as starting at the combination of start date and start transition time" with a UTC offset of the base offset of the zone" (not the rule). We then treat the rule as ending as the combination of end date and end transition time" with a UTC offset of the base offset of the zone + daylight delta of the rule". But that doesn't work in the example above: we end up with a gap of an hour between the two rules.
There's a horrible hack that might fix this: if the end date is on December 31st with an end transition time of 23:59:59 (with any subsecond value), we could ignore daylight savings.
In implementing that, I found a commented out piece of code which did it, which was effectively abandoned in the refactoring described above - so that explains my confusion about why the code had only just stopped working.
With that in place, the data-based unit tests are green.
Now to run the main set of unit tests...
TimeZoneInfo-based unit testsJust to reiterate, I have two types of tests for this code:
- Unit tests based on rules described in a text file. These can be run on any implementation, and always represent the rule data we'd expect to see on Unix". There are currently 16 tests here - specific periods of history for specific time zones.
- Unit tests based on the TimeZoneInfo objects exposed by the BCL on the system we're running on. These include checking every time zone, and ensuring that every transition between 1950 and either 2037 or 2050 (depending on the system, for reasons I won't go into now) is the same between the TimeZoneInfo representation, and the Noda Time representation of the TimeZoneInfo.
The first set of tests is what we've been checking so far - I now need to get the second set of tests working in at least four contexts: .NET Core 3.1 and .NET 6, on both Windows and Linux.
When I started this journey, the tests were working on Windows (both .NET Core 3.1 and .NET 6) and on Linux on .NET Core 3.1. It was only .NET 6 that was failing. Let's start by checking that we haven't broken anything on Windows... yes, everything is still working there. That's pretty unsurprising, given that I've aimed to keep the Windows code path exactly the same as it was before. (There's a potential optimization I can apply using the new BaseUtcOffsetDelta property on .NET 6, but I can apply that later.)
Next is testing .NET Core 3.1 on Linux - this used to work, but I wouldn't be surprised to see problems introduced by the changes... and indeed, the final change I made broke lots of time zones due to trying to add daylight savings to DateTime.MaxValue. That's easily fixed... and with that fix in place there are two errors. That's fine - I'll check those later and add text-file-data-based tests for those two time zones. Let's check .NET 6 first though, which had large numbers of problems before... now we have 14. Definite progress! Those 14 failures seem to fall into two categories, so I'll address those first.
Adding more test data and fixing one problemFirst, I'm going to commit the code I've got. It definitely needs changing, but if I try something that doesn't help, I want to be able to get back to where I was.
Next, let's improve the exception messages thrown by the .NET 6 code. There are two exceptions, that currently look like this:
(For Pacific/Wallis and others)System.InvalidOperationException : Zone recurrence rules have identical transitions. This time zone is broken.(For America/Creston and others)System.ArgumentException : The start Instant must be less than the end Instant (Parameter 'start')
Both have stack traces of code, but that doesn't help me know what the invalid values are, which means I can't easily find the relevant rules to work on.
After a few minutes of work, this is fixed and the output is much more informative:
(For Pacific/Wallis and others)System.InvalidOperationException : Zone recurrence rules have identical transitions. This time zone is broken. Transition time: 0002-12-31T11:45:00Z(For America/Creston and others)System.ArgumentException : The start Instant must be less than the end Instant. start: 1944-01-01T07:00:00Z; end: 1944-01-01T06:01:00Z (Parameter 'start')
The broken rule for Pacific/Wallis is particularly interesting - year 2AD! So let's see what the rules look like in textual form. First let's look at Pacific Wallis
Pacific/Wallis.NET Core 3.1:Base offset = 120001-01-01 - 1900-12-31: Base UTC offset delta: +00:15; Daylight delta: +00; DST starts January 01 at 00:00:00 and ends December 31 at 23:44:391900-12-31 - 2038-01-19: Daylight delta: +00; DST starts December 31 at 23:44:40 and ends January 19 at 15:14:062038-01-19 - 9999-12-31: Daylight delta: +00; DST starts January 19 at 15:14:07 and ends December 31 at 23:59:59.NET 6.0:Base offset = 120001-01-01 - 0001-12-31: Base UTC offset delta: +00:15; Daylight delta: +00; DST starts January 01 at 00:00:00 and ends December 31 at 23:59:59.9990002-01-01 - 1899-12-31: Base UTC offset delta: +00:15; Daylight delta: +00; DST starts January 01 at 00:00:00 and ends December 31 at 23:59:59.9991900-01-01 - 1900-12-31: Base UTC offset delta: +00:15; Daylight delta: +00; DST starts January 01 at 00:00:00 and ends December 31 at 23:44:39.999Noda Time zone intervals:0001-01-01T00:00:00Z - 1900-12-31T11:44:40Z, +12:15:20, +01900-12-31T11:44:40Z - 9999-12-31T23:59:59Z, +12, +0
The first Noda Time zone interval extends from the start of time, and the second one extends to the end of time. I haven't yet decided whether I'll actually try to represent all of this in the regular" kind of test. The offsets shown as +00:15 should actually be +00:15:20, but it looks like .NET doesn't handle sub-minute offsets. That's interesting... I can easily change the Noda Time data to round to expect 12:15 of course.
Both .NET Core 3.1 and .NET 6 have pretty interesting" representations here:
- Why does .NET Core 3.1 have a new rule in 2038? It's no coincidence that the instant being represented is 231 seconds after the Unix epoch, I'm sure... but there's no need for a new rule.
- Why does .NET 6 have one rule for year 1AD and a separate rule for years 2 to 1899 inclusive?
- Why use an offset rounded to the nearest minute, but keep the time zone transition at 1900-12-31T11:44:40Z?
It's not clear to me just from inspection why this would cause the Noda Time conversion to fail, admittedly. But that'll be fun to dig into. Before we do, let's find the test data for America/Creston, around 1944:
America/Creston.NET Core 3.1:Base offset = -71942-02-09 - 1944-01-01: Daylight delta: +01; DST starts February 09 at 02:00:00 and ends January 01 at 00:00:591943-12-31 - 1944-04-01: Daylight delta: +00; DST starts December 31 at 23:01:00 and ends April 01 at 00:00:591944-04-01 - 1944-10-01: Daylight delta: +01; DST starts April 01 at 00:01:00 and ends October 01 at 00:00:591944-09-30 - 1967-04-30: Daylight delta: +00; DST starts September 30 at 23:01:00 and ends April 30 at 01:59:59.NET 6.0:Base offset = -71942-02-09 - 1942-12-31: Daylight delta: +01; DST starts February 09 at 02:00:00 and ends December 31 at 23:59:59.9991943-01-01 - 1943-12-31: Daylight delta: +01; DST starts January 01 at 00:00:00 and ends December 31 at 23:59:59.9991944-01-01 - 1944-01-01: Daylight delta: +01; DST starts January 01 at 00:00:00 and ends January 01 at 00:00:59.9991944-04-01 - 1944-10-01: Daylight delta: +01; DST starts April 01 at 00:01:00 and ends October 01 at 00:00:59.9991967-04-30 - 1967-10-29: Daylight delta: +01; DST starts April 30 at 02:00:00 and ends October 29 at 01:59:59.999Noda Time zone intervals:1942-02-09T09:00:00Z - 1944-01-01T06:01:00Z, -7, +11944-01-01T06:01:00Z - 1944-04-01T07:01:00Z, -7, +01944-04-01T07:01:00Z - 1944-10-01T06:01:00Z, -7, +11944-10-01T06:01:00Z - 1967-04-30T09:00:00Z, -7, +0
Well those are certainly interesting" rules - and I can immediately see why Noda Time has rejected the .NET 6 rules. The third rule starts at 1944-01-01T07:00:00Z (assuming that the 1944-01-01T00:00:00 is in zone standard time of UTC-7) and finishes at 1944-01-01T06:01:00Z (assuming that the 1944-01-01T00:00:59.999 is in daylight time).
Part of the problem is that we've said before that if a rule ends on December 31st at 23:59:59, we'll interpret that as being in standard time instead of being in daylight time... which means that the second rule would finish at 1944-01-01T07:00:00Z - but we genuinely want it to finish at 06:00:00Z, and maybe understand the third rule to mean 1944-01-01T06:00:00Z to 19:44-01-01T06:01:00Z for that last minute of daylight time before we observe standard time until April 1st.
We could do that by adding special rules:
- If a rule appears to end before it starts, assume that the start should be treated as daylight time instead of standard time. (That would make the third rule valid, covering 1944-01-01T06:00:00Z to 19:44-01-01T06:01:00Z.)
- If one rule ends after the next one starts, change its end point to the start of the next one. I currently have the reverse logic to this, changing the start point of the next one instead. That wouldn't help us here. I can't remember exactly why I've got the current logic: I need to add some comments on this bit of code...
Astonishingly, this works, getting us down to 8 errors on .NET 6. Of these, 6 are the same kind of error as Pacific/Wallis, but 2 are unfortunately of the form you created a time zone successfully, but it doesn't give the same results as the original one". Hmm.
Still, let's commit this change and move on to Pacific/Wallis.
Handling Pacific/WallisOnce I'd added the Pacific/Wallis data, those tests passed - which means the problem must lie somewhere in how the results of the rules are interpreted in order to build up a DateTimeZone from the converted rules. That's logic's already in a BuildMap method, which I just need to make internal instead of private. That also contains some code which we're essentially duplicating in the test code (around inserting standard zone intervals if they're missing, and coalescing some zone intervals together). At some point I want to refactor both the production and test code to remove the duplication - but I want to get to working code first.
I'll add a new test, just for Pacific/Wallis (as that's the only test case we've got which is complete from the start to the end of time), and which just constructs the map. I expect it will throw an exception, so I'm not actually going top assert anything about the result yet.
Hmm. It doesn't throw. That's weird. Let's rerun the full time zone tests to make sure we still have a problem at all... yes, it's still failing.
At this point, my suspicion is that some of the code that is duplicated" between production and test code really isn't quite duplicated at all. Debugging the code on Linux is going to be annoying, so let's go about this the old-fashioned way: logging.
I'd expected to be able to log the zone interval for each part of the map... but PartialZoneIntervalMap.GetZoneInterval fails, which is really, really weird. What's even weirder is that the stack trace includes StandardDaylightAlternatingMap - which is only used in the Windows rules.
All my unit tests assume we've recognized that the adjustment rules are from Unix... but the ones for Pacific/Wallis actually look like Windows ones: on .NET 6, they start on January 1st, and end on December 31st.
Let's add a grotty hack: I happen to know that Windows time zone data never has any really old" rules other than ones that start at the beginning of time - if we see anything with a start year that's not 1 and isn't after (say) 1600, that's going to be a Unix rule.
Put that extra condition in, and lo and behold, Pacific/Wallis starts working - hooray!
Let's rerun everything...So, running all the tests on every framework/platform pair that I can easily test, we get:
- Linux, .NET 6: 2 failures - Australia/Broken_Hill and Antarctica/Macquarie
- Linux, .NET Core 3.1: 3 failures - Asia/Dhaka, Australia/Broken_Hill and Antarctica/Macquarie
- Windows, .NET 6: all tests pass
- Windows, .NET Core 3.1: all tests pass
All the remaining failures are of the form the offset is wrong at instant X".
So, joy of joys, we're back to collecting more data and adding more test cases.
First though, I'll undo making a few things internal that didn't actually help in the end. I might redo them later, but I don't need them now. Basically the extra test to create the full map didn't give me any more insight. (There's a bunch of refactoring to do when I've got all the tests passing, but I might as well avoid having more changes than are known to be helpful.)
Going for greenAt this point, I should reveal that I have a hunch. One bit of code I deleted when rewriting the Unix rule to zone interval" conversion code was the opposite of the issue I described earlier of rules which are in daylight savings, but have a DaylightDelta of zero. The code I deleted basicaly said If the time zone says it's not in daylight savings, but DaylightDelta is non-zero, then treat it as zero anyway." So I'm hoping that's the issue, but I want to get the test data first. I'm hoping that it's the same for all three time zones that are having problems though. We'll start with Australia/Broken_Hill, which is failing during 1999. Dumping the rules in .NET 6 and .NET Core 3.1 under Linux, and looking at the Noda Time tzvalidate page, I get:
Base UTC offset: 09:30.NET Core 3.1:1998-10-25 - 1999-03-28: Daylight delta: +01; DST starts October 25 at 02:00:00 and ends March 28 at 02:59:591999-03-28 - 1999-10-31: Daylight delta: +00; DST starts March 28 at 02:00:00 and ends October 31 at 01:59:591999-10-31 - 1999-12-31: Daylight delta: +01; DST starts October 31 at 02:00:00 and ends December 31 at 23:59:591999-12-31 - 2000-03-26: Daylight delta: +01; DST starts December 31 at 23:00:00 and ends March 26 at 02:59:59.NET 6:1999-01-01 - 1999-03-28: Daylight delta: +01; DST starts January 01 at 00:00:00 and ends March 28 at 02:59:59.9991999-10-31 - 1999-12-31: Daylight delta: +01; DST starts October 31 at 02:00:00 and ends December 31 at 23:59:59.9991999-12-31 - 1999-12-31: Daylight delta: +01; DST starts December 31 at 23:00:00 and ends December 31 at 23:59:59.9992000-01-01 - 2000-03-26: Daylight delta: +01; DST starts January 01 at 00:00:00 and ends March 26 at 02:59:59.999Noda Time:1998-10-24T16:30:00Z - 1999-03-27T16:30:00Z, +09:30, +011999-03-27T16:30:00Z - 1999-10-30T16:30:00Z, +09:30, +001999-10-30T16:30:00Z - 2000-03-25T16:30:00Z, +10:30, +01
Annoyingly, my test data parser doesn't handle partial-hour base UTC offsets at the moment, so I'm going to move on to Antarctica/Macquarie - I'll put Broken Hill back in later if I need to. The text format of Broken Hill would be:
Australia/Broken_HillBase offset = 09:30---1998-10-25 - 1999-03-28: Daylight delta: +01; DST starts October 25 at 02:00:00 and ends March 28 at 02:59:591999-03-28 - 1999-10-31: Daylight delta: +00; DST starts March 28 at 02:00:00 and ends October 31 at 01:59:591999-10-31 - 1999-12-31: Daylight delta: +01; DST starts October 31 at 02:00:00 and ends December 31 at 23:59:591999-12-31 - 2000-03-26: Daylight delta: +01; DST starts December 31 at 23:00:00 and ends March 26 at 02:59:59---1999-01-01 - 1999-03-28: Daylight delta: +01; DST starts January 01 at 00:00:00 and ends March 28 at 02:59:59.9991999-10-31 - 1999-12-31: Daylight delta: +01; DST starts October 31 at 02:00:00 and ends December 31 at 23:59:59.9991999-12-31 - 1999-12-31: Daylight delta: +01; DST starts December 31 at 23:00:00 and ends December 31 at 23:59:59.9992000-01-01 - 2000-03-26: Daylight delta: +01; DST starts January 01 at 00:00:00 and ends March 26 at 02:59:59.999---1998-10-24T16:30:00Z - 1999-03-27T16:30:00Z, +09:30, +011999-03-27T16:30:00Z - 1999-10-30T16:30:00Z, +09:30, +001999-10-30T16:30:00Z - 2000-03-25T16:30:00Z, +10:30, +01
However, let's have a look at Antarctica/Macquarie, which is broken in 2009. Here's the data:
Antarctica/MacquarieBase UTC offset: +10.NET Core 3.1:2008-10-05 - 2009-04-05: Daylight delta: +01; DST starts October 05 at 02:00:00 and ends April 05 at 02:59:592009-04-05 - 2009-10-04: Daylight delta: +00; DST starts April 05 at 02:00:00 and ends October 04 at 01:59:592009-10-04 - 2009-12-31: Daylight delta: +01; DST starts October 04 at 02:00:00 and ends December 31 at 23:59:592009-12-31 - 2011-04-03: Daylight delta: +01; DST starts December 31 at 23:00:00 and ends April 03 at 02:59:59.NET 6.0:2008-10-05 - 2008-12-31: Daylight delta: +01; DST starts October 05 at 02:00:00 and ends December 31 at 23:59:59.9992009-01-01 - 2009-04-05: Daylight delta: +01; DST starts January 01 at 00:00:00 and ends April 05 at 02:59:59.9992009-10-04 - 2009-12-31: Daylight delta: +01; DST starts October 04 at 02:00:00 and ends December 31 at 23:59:59.9992009-12-31 - 2009-12-31: Daylight delta: +01; DST starts December 31 at 23:00:00 and ends December 31 at 23:59:59.9992010-01-01 - 2010-12-31: Daylight delta: +01; DST starts January 01 at 00:00:00 and ends December 31 at 23:59:59.9992011-01-01 - 2011-04-03: Daylight delta: +01; DST starts January 01 at 00:00:00 and ends April 03 at 02:59:59.999Noda Time:2008-10-04T16:00:00Z - 2009-04-04T16:00:00Z +10, +012009-04-04T16:00:00Z - 2009-10-03T16:00:00Z +10, +002009-10-03T16:00:00Z - 2011-04-02T16:00:00Z +10, +01
(The .NET 6 data needs to go as far as 2011 in order to include all the zone intervals for 2009, because there were no changes in 2010.)
Good news! The test fails... but only in .NET Core 3.1.
(A few days later... this blog post is being written in sporadic bits of spare time.)
Okay, let's check I can still reproduce this - in .NET 6 on Linux, BclDateTimeZone test that converts a TimeZoneInfo to a BclDateTimeZone fails for Antarctica/Macquarie because it gives the wrong offset at 2009-10-04T00:00:00Z - the TimeZoneInfo reports +11, and the BclDateTimeZone reports +10. But the unit test for the .NET 6 data apparently gives the correct ZoneInterval. Odd.
Again, this is tricky to debug, so I'll add some logging. Just a simple new" test that logs all of the zone intervals in the relevant period. The results are:
Australian Eastern Standard Time: [2008-04-05T16:00:00Z, 2008-10-04T16:00:00Z) +10 (+00)Australian Eastern Daylight Time: [2008-10-04T16:00:00Z, 2009-04-04T16:00:00Z) +11 (+01)Australian Eastern Standard Time: [2009-04-04T16:00:00Z, 2009-12-31T13:00:00Z) +10 (+00)Australian Eastern Daylight Time: [2009-12-31T13:00:00Z, 2011-04-02T16:00:00Z) +11 (+01)Australian Eastern Standard Time: [2011-04-02T16:00:00Z, 2011-10-01T16:00:00Z) +10 (+00)Australian Eastern Daylight Time: [2011-10-01T16:00:00Z, 2012-03-31T16:00:00Z) +11 (+01)
It looks like we're not adding in the implicit standard time zone interval between April and October 2009. This is code that I'm aiming to deduplicate between the production code and the rule-data-oriented unit tests - it looks like the unit test code is doing the right thing, but the production code isn't.
(In the process of doing this, I've decided to suppress warning CA1303 - it's completely useless for me, and actively hinders simple debug-via-console-logging.)
Adding extra logging to the BuildMap method, it looks like we've already lost the information by the time we get there: there's no sign of the October 2009 date anywhere. Better look further back in the code...
... and immediately spot the problem. This code, intended to handle overlapping rules:
convertedRules[i - 1] = convertedRules[i].WithEnd(convertedRules[i].Start);
... should be:
convertedRules[i - 1] = convertedRules[i - 1].WithEnd(convertedRules[i].Start);
Again, that's code which isn't covered by the rule-data-oriented unit tests. I'm really looking forward to removing that duplication. Anyway, let's see what that fix leaves us with... oh. It hasn't actually fixed it. Hmm.
Ha. I fixed it in this blog post but not in the actual code. So it's not exactly a surprise that the tests were still broken!
Having actually fixed it, now the only failing test in .NET 6 on Linux is the one testing the .NET Core 3.1 data for Antarctica/Macquarie. Hooray! Running the tests for .NET Core 3.1, that's also the only failing test. The real time zone seems to be okay. That's odd... and suggests that my test data was incorrectly transcribed. Time to check it again... no, it really is that test data. Hmm. Maybe this time there's another bug in the code that's intended to be duplicated between production and tests, but this time with the bug in the test code.
Aha... the .NET Core 3.1 test code didn't have the first fix up overlapping rules" code that's in the .NET 6 tests. The circumstances in which that fix-up is needed happen much more rarely when using the .NET Core 3.1 rules - this is the first time we'd needed it for the rule-data-oriented tests, but it was happening unconditionally in the production code. So that makes sense.
Copy that code (which now occurs three times!) and the tests pass.
All the tests are green, across Windows and Linux, .NET Core 3.1 and .NET 6.0 - wahoo!
Time for refactoringFirst things first: commit the code that's working. I'm pretty reasonable at refactoring, but I wouldn't put it past myself to mess things up.
Okay, let's try to remove as much of the code in the tests as possible. They should really be pretty simple. It's pretty easy to extract the code that fixes up overlapping adjustment rules - with a TODO comment that says I should really add a test to make sure the overlap is an expected kind (i.e. by exactly the amount of daylight savings, which should be the same for both rules). I'll add that later.
The part about coalescing adjacent intervals is trickier though - that's part of a process creating a full" zone interval map, extending to the start and end of time. It's useful to do that, but it leaves us with a couple of awkward aspects of the existing test data. Sometimes we have DST adjustment rules at the start or end of the .NET 6 data just so that the desired standard time rule can be generated.
In the tests we just committed, we accounted for that separately by removing those extra" rules before validating the results. It's harder to do that in the new tests, and it goes against the aim of making the tests as simple as possible. Additionally, if the first or last zone interval is a standard time one, the create full map" code will extend those intervals to the start of time, which isn't what we want either.
Rather than adding more code to handle this, I'll just document that all test data must start and end with a daylight zone interval - or the start/end of time. Most of the test data already complies with this - I just need to add a bit more information for the others.
Interestingly, while doing this, I found that there's an odd discrepancy between data sources for Europe/Prague in 1945 in terms of when daylight savings started:
- TimeZoneInfo says April 2nd in one rule, and then May 8th in another
- Noda Time (and tzvalidate, and zdump) says April 2nd
- timeanddate.com says April 8th
Fortunately, specifying both of the rules from TimeZoneInfo ends up with the tests passing, so I'll take that.
With those data changes in, everything's nicer and green - so let's commit again.
Next up, there's a bit of rearranging of the test code itself. Literally just moving code around, mostly moving it into the two nested helper classes.
Again, run all the tests - still green, so let's commit again. (I'm planning on squashing all of these commits together by the end, of course.)
Next there's a simplification option which I'd noted before but never found time to implement - just removing a bit of redundancy. While fixing that, I've noticed we've got IZoneIntervalMap and IZoneIntervalMapWithMinMax... if we could add min/max offset properties to IZoneIntervalMap, we could simplify things further. I've just added a TODO for this though, as it's a larger change than I want to include immediately. Run tests, commit on green.
When comments become more than commentsNow for some more comments. The code I've got works, but the code to handle the corner cases in BclAdjustmentRule.ConvertUnixRuleToBclAdjustmentRule isn't commented clearly. For every case, I'm going to have a comment that:
- Explains what the data looks like
- Explains what it's intended to mean
- Gives a sample zone + framework (ideally with a date) so I can look at the data again later on
Additionally, the code that goes over a set of converted Unix rules and fixes up the end instant for overlapping rules needs:
- More comments including an example
- Validation to ensure the fix-up only occurs in an expected scenario
- A test for that validation (with deliberately broken rules)
When trying to do this, I've found it hard to justify some of the code. It's all just a bit too hacky. With tests in place that can be green, I've tried to improve things - in particular, there was some code to handle a rule ending at the very end of the year, which changed the end point from daylight time to standard time. That feels like it's better handled in the fix-up" code... but even that's really hard to justify.
What I can do is leave the code there with some examples of what fails. I'm still hoping I can simplify it more later though.
A new fly in the ointmentIn the course of doing this, I've discovered one additional tricky aspect: in .NET 6, the last adjustment rule can be a genuine alternating one rather than a fixed one. For example, Europe/London finishes with:
2037-10-25 - 9999-12-31: Daylight delta: +01; DST starts Last Sunday of March; 01:00:00 and ends Last Sunday of October; 02:00:00
Currently we don't take that into account, and that will make life trickier. Sigh. That rule isn't too hard to convert, but it means I'm unlikely to get there today after all.
It shouldn't be too hard to test this though: we currently have this line of code in BclDateTimeZoneTest:
// Currently .NET Core doesn't expose the information we need to determine any DST recurrence// after the final tzif rule. For the moment, limit how far we check.// See https://github.com/dotnet/corefx/issues/17117int endYear = TestHelper.IsRunningOnDotNetCoreUnix ? 2037 : 2050;
That now needs to be on .NET Core 3.1, use 2037; on .NET 6 use 2050". With that change in place, I expect the tests to fail. I've decided I won't actually implement that in the first PR; let's get all the existing tests working first, then extend them later.
Let's get it merged...Even though there's more work to do, this is much better than it was.
It's time to get it merged, and then finish up the leftover work. I might also file an issue asking for Microsoft to improve the documentation and see if they're able to provide sample code that makes sense of all of this...